Starting with a disclaimer:
In this tutorial I won't teach you Java, nor will we have time to cover the Bukkit API. There is a good guide on how to start with Java, and there are good video classes or books that you can read to start with. You will need a jdk 7 or higher, a working IDE (integrated development environment), a compiled version of Spigot and being able to run that server.
This tutorial is for you if you are familiar with the Java syntax and OOP (object oriented programming) methodology, are aware of the basic structure of a Bukkit plugin and want to dive into plugin development with the help of MassiveCore.
Preparation
To work, modify and test the classes shown in this tutorial you need to download MassiveCore and MassiveBooks either by using git or downloading the zip from github. MassiveCore only needs the Spigot jar as a dependency and MassiveBooks needs the spigot jar and the MassiveCore project as dependencies.
A few links worth looking at:
For this tutorial we will be looking at and working with the plugin MassiveBooks. You might have read the Page on MassiveCraft.com, it will link the code you'll be seeing with what we as players can interact with.
MassiveBooks is a "fairly" simple, yet fully equipped MassivePlugin. It makes use of many of the components and thus is a good example.
Chapter 0 - The Main class
Bukkit plugins need atleast two things: A plugin.yml and a main class (class extending javaPlugin). The plugin.yml is also part of all MassivePlugins, and by convention our main classes are called the same as the project.
In our case the main class is located here:
The class MassiveBooks extends MassivePlugin. One of our tech standards is to use Singletons when applicable. In case you didn't know what a singleton is: At any time there is only one instance of said class present. This is usefull in our case here, as we don't want two instances of our main class.
Bukkit plugins have onEnable and onDisable methods, MassivePlugins makes this step a little easier for you with the onEnableInner method. The super class MassivePlugin saves when you started and when you stopped to enable your plugin, so you can see how long it takes.
Noticably, the activate method is what get's the stone rolling. Here we instantiate/activate classes so they are starting to work. We activate Collections (data storage), Commands and Engines (Event Listeners) and anything else we might need for the plugin to function properly.
In the further chapters of this guide you will learn about the other important parts of a MassivePlugin and eventually about substantial classes of MassiveCore.
Chapter 1 - Collections
To store data persistently (over restarts) we came up with the concept of Entities and Collections.
For example, let's look at the MBookColl:
It is a Singleton and extends Coll<MBook>. From that we know, it stores and manages all the MBook objects and files. Also, in your mstore folder (located in your directory you run the spigot server), a folder called massivebooks_mbook will appear and in it are the files representing the MBook objects.
MBook extends Entity. What is so special about it? All the fields in a class extending Entity are automatically persistent, as long as you put them in the load. You as a developer don't need to take care of storing/fetching data, the Collections handles that for you. To notify the collection that a value has changed code wise (through a setter for example), you must call changed. If you change a file directly, the changes will get loaded to the server in a matter of seconds.
This is the magic behind our powerful configuration system, where we can adapt config values on runtime and they will get applied within seconds rather after a restart.
Try it out
If you want to play around, add some extra field to MBook, don't forget to put them into the load method and see how it reflects in a file entry on your local folder and will persist over restarts.
Add getters and setters, make sure to call changed or see what happens if you forget to do that.
In this tutorial I won't teach you Java, nor will we have time to cover the Bukkit API. There is a good guide on how to start with Java, and there are good video classes or books that you can read to start with. You will need a jdk 7 or higher, a working IDE (integrated development environment), a compiled version of Spigot and being able to run that server.
This tutorial is for you if you are familiar with the Java syntax and OOP (object oriented programming) methodology, are aware of the basic structure of a Bukkit plugin and want to dive into plugin development with the help of MassiveCore.
Preparation
To work, modify and test the classes shown in this tutorial you need to download MassiveCore and MassiveBooks either by using git or downloading the zip from github. MassiveCore only needs the Spigot jar as a dependency and MassiveBooks needs the spigot jar and the MassiveCore project as dependencies.
A few links worth looking at:
For this tutorial we will be looking at and working with the plugin MassiveBooks. You might have read the Page on MassiveCraft.com, it will link the code you'll be seeing with what we as players can interact with.
MassiveBooks is a "fairly" simple, yet fully equipped MassivePlugin. It makes use of many of the components and thus is a good example.
Chapter 0 - The Main class
Bukkit plugins need atleast two things: A plugin.yml and a main class (class extending javaPlugin). The plugin.yml is also part of all MassivePlugins, and by convention our main classes are called the same as the project.
In our case the main class is located here:
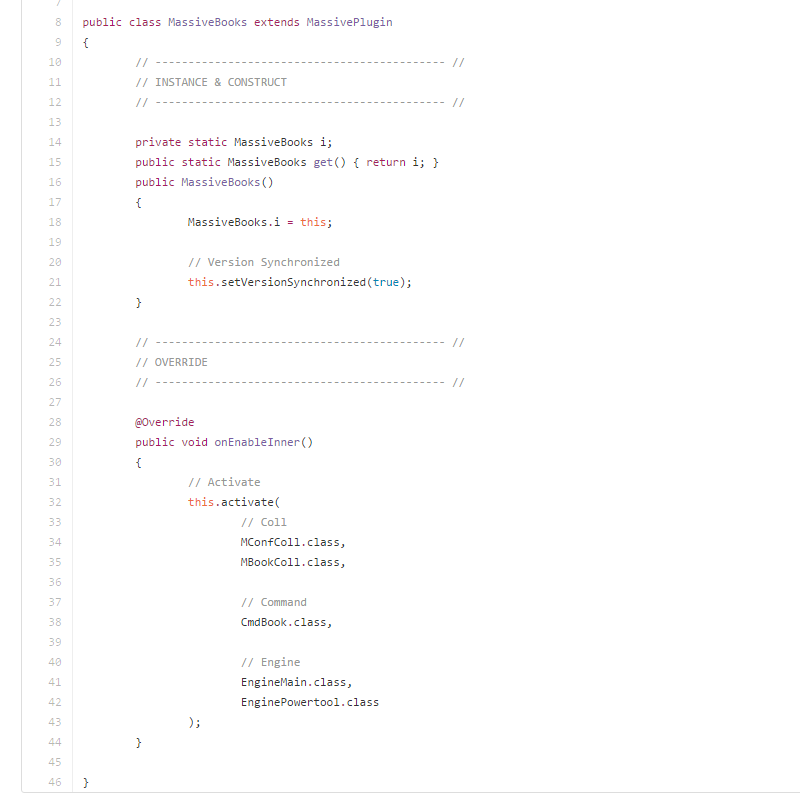
The class MassiveBooks extends MassivePlugin. One of our tech standards is to use Singletons when applicable. In case you didn't know what a singleton is: At any time there is only one instance of said class present. This is usefull in our case here, as we don't want two instances of our main class.
Bukkit plugins have onEnable and onDisable methods, MassivePlugins makes this step a little easier for you with the onEnableInner method. The super class MassivePlugin saves when you started and when you stopped to enable your plugin, so you can see how long it takes.
Noticably, the activate method is what get's the stone rolling. Here we instantiate/activate classes so they are starting to work. We activate Collections (data storage), Commands and Engines (Event Listeners) and anything else we might need for the plugin to function properly.
In the further chapters of this guide you will learn about the other important parts of a MassivePlugin and eventually about substantial classes of MassiveCore.
Chapter 1 - Collections
To store data persistently (over restarts) we came up with the concept of Entities and Collections.
For example, let's look at the MBookColl:
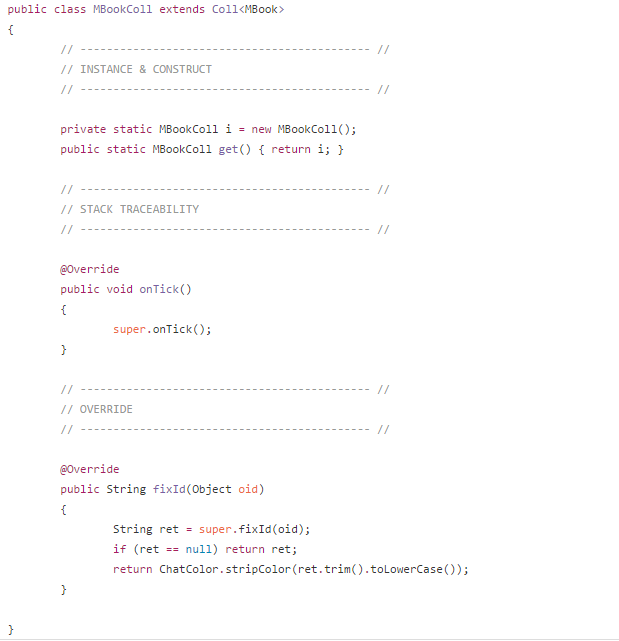
It is a Singleton and extends Coll<MBook>. From that we know, it stores and manages all the MBook objects and files. Also, in your mstore folder (located in your directory you run the spigot server), a folder called massivebooks_mbook will appear and in it are the files representing the MBook objects.
MBook extends Entity. What is so special about it? All the fields in a class extending Entity are automatically persistent, as long as you put them in the load. You as a developer don't need to take care of storing/fetching data, the Collections handles that for you. To notify the collection that a value has changed code wise (through a setter for example), you must call changed. If you change a file directly, the changes will get loaded to the server in a matter of seconds.
This is the magic behind our powerful configuration system, where we can adapt config values on runtime and they will get applied within seconds rather after a restart.
Try it out
If you want to play around, add some extra field to MBook, don't forget to put them into the load method and see how it reflects in a file entry on your local folder and will persist over restarts.
Add getters and setters, make sure to call changed or see what happens if you forget to do that.